
How to Use GraphQL with AngularJS for Better Data Fetching
Feb 12
3 min read
0
1
0
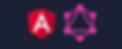
Modern web applications require efficient data fetching mechanisms to improve performance and user experience. GraphQL has emerged as a powerful alternative to REST APIs, offering precise data retrieval with minimal over-fetching. When combined with AngularJS, a dynamic JavaScript framework, GraphQL enhances frontend development by providing seamless data management.
For businesses looking to optimize their web applications, hiring the right expertise is crucial. If you want to implement GraphQL effectively, you might consider hiring professionals with relevant skills. You can hire AngularJS developers to integrate GraphQL seamlessly into your projects. Additionally, businesses seeking cost-effective solutions may explore IT project outsourcing for GraphQL-empowered AngularJS applications.
This guide will cover:
Understanding GraphQL and its advantages
Setting up GraphQL with AngularJS
Fetching and manipulating data with GraphQL
Best practices for performance optimization
Understanding GraphQL and Its Advantages

GraphQL is a query language for APIs that provides a flexible and efficient alternative to REST. It allows clients to request only the data they need, reducing unnecessary data transfer. Here’s why GraphQL stands out:
Precise Data Retrieval: Unlike REST, which often returns fixed structures, GraphQL lets clients specify exactly what they need.
Reduced Network Requests: Multiple related data entities can be fetched in a single request.
Strongly Typed Schema: GraphQL APIs define a strict schema, improving validation and maintainability.
Real-Time Capabilities: GraphQL supports real-time data updates via subscriptions.
Better Developer Experience: Its self-documenting nature makes API development easier.
To leverage these benefits effectively, companies often hire AngularJS developers who understand how to integrate GraphQL into web applications.
Setting Up GraphQL with AngularJS
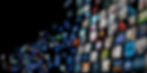
1. Prerequisites
Before diving into GraphQL integration, ensure you have:
Node.js and npm installed
An AngularJS application set up
A GraphQL API server ready
You can choose a backend like Apollo Server, Hasura, or a custom-built GraphQL service.
2. Installing Required Packages
To use GraphQL with AngularJS, install the necessary dependencies:
npm install apollo-angular graphql @apollo/client
Apollo Client is the most popular GraphQL client, providing an easy way to interact with GraphQL APIs.
3. Configuring Apollo Client
Set up Apollo Client in your AngularJS application:
import { ApolloClient, InMemoryCache } from '@apollo/client';
const client = new ApolloClient({
uri: 'https://your-graphql-endpoint.com/graphql',
cache: new InMemoryCache()
});
This configuration establishes a connection to your GraphQL API.
Fetching Data with GraphQL in AngularJS
1. Writing GraphQL Queries
Create a GraphQL query to fetch user data:
import { gql } from '@apollo/client';
const GET_USERS = gql`
query {
users {
id
name
email
}
}
`;
2. Executing Queries in AngularJS Components
Integrate the GraphQL query into an AngularJS component:
app.controller('UserController', function($scope) {
client.query({ query: GET_USERS })
.then(response => {
$scope.users = response.data.users;
$scope.$apply();
})
.catch(error => console.error(error));
});
This fetches user data and updates the scope accordingly.
Mutating Data with GraphQL
GraphQL allows creating, updating, and deleting data through mutations.
1. Writing a Mutation
Here’s an example mutation for adding a new user:
const ADD_USER = gql`
mutation AddUser($name: String!, $email: String!) {
addUser(name: $name, email: $email) {
id
name
email
}
}
`;
2. Executing the Mutation
client.mutate({
mutation: ADD_USER,
variables: { name: 'John Doe', email: 'johndoe@example.com' }
})
.then(response => console.log('User added:', response.data.addUser))
.catch(error => console.error(error));
Optimizing Performance in GraphQL + AngularJS Applications
To ensure your GraphQL-powered AngularJS app runs smoothly, consider these best practices:
1. Caching Responses
Apollo Client includes an in-memory cache that prevents redundant network requests. Always enable caching:
const client = new ApolloClient({
uri: 'https://your-graphql-endpoint.com/graphql',
cache: new InMemoryCache()
});
2. Using Pagination
For large datasets, implement pagination to reduce payload size:
const GET_PAGINATED_USERS = gql`
query GetUsers($limit: Int!, $offset: Int!) {
users(limit: $limit, offset: $offset) {
id
name
email
}
}
`;
3. Subscriptions for Real-Time Updates
Enable real-time data updates using GraphQL subscriptions:
const NEW_USER_SUBSCRIPTION = gql`
subscription {
newUser {
id
name
email
}
}
`;
Benefits of Using GraphQL in IT Project Outsourcing
For companies engaged in IT project outsourcing, GraphQL provides:
Improved API Flexibility: Clients get only the data they need.
Faster Development Cycles: Self-documenting APIs reduce communication gaps.
Better Performance: Reduced over-fetching leads to efficient network usage.
Organizations that hire AngularJS developers skilled in GraphQL can benefit from streamlined development and enhanced frontend performance.
Conclusion
GraphQL and AngularJS together create a powerful combination for efficient data fetching. By leveraging GraphQL’s query capabilities, AngularJS applications can be more performant, flexible, and user-friendly.
Businesses looking to optimize their web solutions should consider IT project outsourcing or hiring AngularJS developers to ensure smooth integration and maximum benefits. By following best practices such as caching, pagination, and subscriptions, developers can build scalable and high-performing applications.
Start implementing GraphQL with AngularJS today to revolutionize your data-fetching strategy!